QR Code or the Quick Response Code is a square-shaped matrix barcode that contains data encoded within it. QR Codes can be generated using JavaScript or jQuery too and in this article, we’ll concentrate on how to generate QR code using jquery. QR Codes have become very common in recent times especially among portable devices like tablets and smartphones with cameras. It has made it easy to store different types of info in a small box type barcode. I generally find it on products that have their web address stored in the QR Code and as soon as I scan it, my smartphone shows me the web address encoded in it and also shows me a button to go to the embedded URL. It can be found on some ID cards too with data stored in different formats like XML or Text etc. Basically, QR Codes have become very handy and have become very common over the web.
With QR Codes spreading over the web, many clients ask for QR Codes to be shown on websites so that users can either print it, scan it on their device or save it as an image for future reference. With this advancement, one general question everybody has is “How do we generate a QR Code dynamically ?” Well, there are different ways of doing it but we’ll look into generating QR Code using jQuery.
Did you Know ?
The word “QR Code” is registered trademark of DENSO WAVE INCORPORATED in countries Japan, United States of America, Australia and Europe.
Generating QR Code using jQuery
Well, jQuery does not have an in-built way to do it but Jerome Etienne has written a jQuery plugin which actually is a wrapper around the library written by Kazuhiko Arase (who wrote it in different programming languages). Both were released under an MIT license.
Steps to generate QR code using jQuery
Following are the steps to generate QR code using jQuery. Depending on how you are developing the page, you might have to include the plugins on your page differently. However, the concept remains the same.
Step 1.
First of all, you’ll have to download the jQuery core file. You can do that from jQuery’s official website.
OR
You can also link to the jQuery file hosted on google Or any other jQuery CDN. You might want to install the latest jquery package using npm if you are using npm in your project to manage packages.
Step 2.
Secondly, download and include the jquery.qrcode.min.js file from the GIT Hub page.
Now your code might look similar to the following :
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script type="text/javascript" src="jquery.qrcode.min.js"></script>
Step 3.
Thirdly, create a DOM element like a DIV or a span, etc to use as a container for the QR Code generated. We’ll use a DIV in our example.
<div id="qrcodeholder"> </div>
Step 4.
Lastly, invoke the qrcode plugin function with the parameters and the data you want to encode in the QR Code.
The parameters for the jQuery qrcode plugin include the text or data you want to encode, the width and height of the QR Code generated, and the rendering mode. The render param has two values namely “canvas” and “table”. Table value can be used with browsers that don’t support HTML5 canvas.
//Put this code in your js file or wrap it within the <script></script> tags
//Wrap it within $(document).ready() to invoke the function after DOM loads.
$(document).ready(function(){
$('#qrcodeholder').qrcode({
text : "https://www.moreonfew.com/generate-qr-code-using-jquery",
render : "canvas", // 'canvas' or 'table'. Default value is 'canvas'
background : "#ffffff",
foreground : "#000000",
width : 150,
height: 150
});
});
The above code generates the following QR Code :
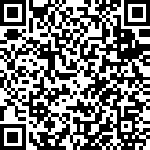
Isn’t it very easy to generate the QR Code using jQuery? Likewise, you can also generate QR Code using JavaScript too. Find the appropriate plugin that you can use. However, please do check if the plugin you are using is maintained and updated by its author. Hope you liked this article. Also please do like us on Facebook. You may also follow us on Twitter for interesting Tips and Tricks. Also please do share the post with your friends if you like it. Share your thoughts.
thank you very much, for Tutorial
apparently, it is very easy to add QR codes.
Best Regards
Yes, its really great , crisp and clear..
Thanx
@Arunbala , I’m happy to know that you liked it.
Are there any options to have the output to be a string of 0’s and 1’s?
@Joe Murphy can you be a little more specific?
Something like:
111111101001101111111|100000100110101000001|101110101110101011101|…….
I use the results to make an eps file.
@Joe Murphy, you can try passing the data as text in options. But there is a max length that QR codes can support (upto 2953 for binary). P.S : I haven’t tried passing binary yet though 🙂 .
Hi — I don’t want to pass binary text as input but want to receive an output of a text string of 0′ and 1’s. I tried ‘text’ instead of ‘table’ or ‘canvas’ for the render but it gave back same as ‘table’. If I can get text output as 0’s and 1’s I can use javascript to write an eps file.
e.g.,
%!PS-Adobe-2.0 EPSF-1.2
%%BoundingBox: 0 0 115 115
%%Creator: Joe Murphy
%%Title: eps QRcode with background transparency
%%CreationDate: 09-13-2013
%%EndComments
/rgb { {255 div}forall setrgbcolor } def % hex color to ps rgb
% rgb 0 0 115 dup rectfill % uncomment for yellow background
5 dup scale % size of block in pts
1 dup translate % offset blocks for padding
rgb % foreground color/background is transparent
/x 0 store /y 20 store % start in upper left corner, move right then down
(111111101111101111111|100000101010101000001|101110101000001011101|101110101111001011101|101110100000101011101|100000101101001000001|111111101010101111111|000000000111100000000|110011100101000101111|101011000011100101100|101000101011001110001|001111010100011011111|011111111110111000001|000000001010111000111|111111100110110001010|100000101001100101010|101110101111001110111|101110100001100101011|101110100101001111100|100000101100011010110|111111101100111000111) % binary string of blocks
{dup % need two values for two conditional choices
49 eq {x y 1 1 rectfill} if % only fill/paint foreground if 1
124 eq {/x 0 store /y y 1 sub store}{/x x 1 add store} ifelse % Check for |
} forall % do the whole string
%%EOF
The previous example left out ffff00 and 000000 before rgb where the hex numbers are in between angled brackets or lt, gt signs.
isn’t it easier without the use of jquery?
It depends, if you are new to jQuery you’ll find the native JavaScript approach to be easier else jQuery approach is way easier. Even the PHP and .NET versions of the QR Code generator provided by the original author is good.
Very simple and easily understandable. Thanks
Dear admin,
How can i convert above barcode to doc file in PHP?
Thanks
Tenh
Hi Tenh,
I will be able to help you out only with the JavaScript or jQuery version of the QR Code generator. Thanks for visiting the site.
Hello,admin
You should remove an extra braces of document.ready() function in Step 4.
Thanks Sandip for pointing that out. It has been fixed.
You are most welcome.
It is very helpful for newbie and easy to understand. It help me a lot.
Good job. Keep going.
Thanks for your appreciate.
Super You are Great Keep Updating…
Qrcode generated successfully, If I resized into small size regarding my UI, Couldn’t able to read the qrcode from my mobile application.
Is there anyway way to create the qrcode with a limited size?
Already used height & width as like above description in this page, Still no luck 🙁