You might be facing an “Attempted import error: ‘Switch’ is not exported from ‘react-router-dom’ ” error if you are using the react-router-dom package version 6. This error is caused due to using the older switch syntax of the react-router-dom. From version 6 onwards, the react-router-dom has replaced “Switch” with “Routes”. Let us take a look at how to solve the ‘Switch’ is not exported from ‘react-router-dom’ error.
What causes the ‘Switch’ is not exported from ‘react-router-dom’ error in Reactjs?
As I mentioned earlier, the cause of the ‘Switch’ is not exported from ‘react-router-dom’ error mostly is because you are using v6 or above of react-router-dom whereas the syntax you are using is still the older one. In react-router-dom v6 onwards, the “Switch” is replaced by “Routes”.
An Example of code that might throw the error:
import React from 'react';
import './main.css';
import NavBar from './components/navbar.js';
import HomePage from './components/pages/homepage.js';
import Footer from './components/footer.js';
import { BrowserRouter as Router, Switch, Route, Link } from 'react-router-dom';
function App() {
return (
<Router>
<div className="main-wrapper">
<NavBar />
<Switch>
<Route path="/home" component={HomePage} />
</Switch>
<Footer />
</div>
</Router>
);
}
export default App;
And the package.json might have the following
"dependencies": {
...
"react-router-dom": "^6.2.1" // Version 6 of React
},
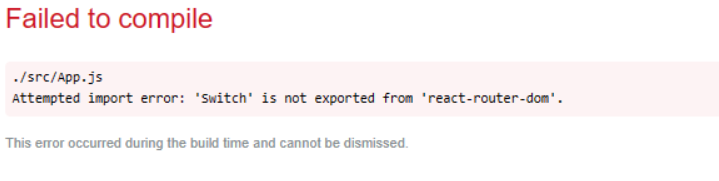
In the above code we can notice that the code is using <Switch> , whereas the package.json says that we are using v6 of react-router-dom.
There are 2 ways we can solve the ‘Switch’ is not exported from ‘react-router-dom’ error in ReactJs.
- Solution 1 : Upgrade the react-router-dom and the related code to the latest version
- Solution 2 : Downgrade react-router-dom to V5 so that the code understands the <Switch> Statement.
Lets us take a look at both the solutions.
How to solve ‘Switch’ is not exported from ‘react-router-dom’ error in ReactJs? Solution 1
In order to solve the ‘Switch’ is not exported from ‘react-router-dom’ error in ReactJs, we need to update the syntax of the routes defined. From version 6 onwards, the react-router-dom has replaced “Switch” with “Routes”. And hence we need to update the syntax accordingly. Let us take a look at the steps to solve the ‘Switch’ is not exported from ‘react-router-dom’ error.
Step 1. Upgrade to React 16.8+ and react-router-dom v6+
Firstly you would have to upgrade to react version 16.8 or above version as react-router-dom version 6 makes heavy use of React hooks.
Secondly, upgrade to react-router-dom v6+ using the following command if you are already not using the latest version or V6:
npm install react-router-dom
You can also use the @latest keyword to install the latest version of the package
Step 2: Update the react-router-dom import statement.
Now that you have React 16.8+ and react-router-dom v6+, you should update the Router’s syntax. Firstly we need to update the import statement to import “Routes” instead of “Switch”.
So, Update the import statement like the following
//import { Switch, Route } from "react-router-dom";
//to
import { Routes ,Route } from 'react-router-dom';
Step 3: Upgrade the syntax and replace “Switch” with “Routes” and “component” with “element’
The third step would be to replace the “Switch” with “Routes” and the “component” with “element” as shown in the example.
So, replace the <Switch> with <Routes> and component attribute to element, like in the following example
// <Switch>
// <Route path="/home" component={HomePage} />
// </Switch>
//to
<Routes>
<Route path="/home" element={<HomePage/>} />
</Routes>
Notice that the component={HomePage} too got updated to element={<HomePage/>} as per the new syntax. You can read more about the process to update react-router-dom from v5 to v6 at https://reactrouter.com/docs/en/v6/upgrading/v5#upgrade-to-react-router-v6 .
An alternative way to fix the ‘Switch’ is not exported from ‘react-router-dom’. Solution 2
There is an alternative way to fix the ‘Switch’ is not exported from ‘react-router-dom’ error too. As we discussed earlier, the error is caused due to using react-router-dom version 6+ and using the older syntax of Router. So if you don’t have the time to update all the syntax, the alternative would be to downgrade the react-router-dom package to version 5.2.0 or 5.3.0.
You can downgrade to react-router-dom version 5.2.0 by first uninstalling the package version installed and then installing the v5.2.0. You can do so by using the following commands:
npm uninstall react-router-dom
then use the following command to install a specific version of react-router-dom
npm install [email protected]
This way you would not have to worry about upgrading react-router-dom’s syntax and avoid all the hassle temporarily. On the long run I would recommend you to always upgrade to the latest version of the package . This can be useful to fix the issue ” ‘Routes’ is not exported from ‘react-router-dom’ “, which can be caused because you are using older version of react-router-dom but newer version of code.
To conclude, I can say that to solve the ‘Switch’ is not exported from ‘react-router-dom’ error, the best way is to upgrade to react-router-dom v6 or above while updating the syntax too. However, alternatively, if you don’t want to update to react-router-dom version 6, you can also instead downgrade to version 5.2.0 , in which case, you need not update the syntax code too.