Many times we would have come across scenarios where we wanted to create a unique string in JavaScript that we could use as a unique ID. The obvious thought we would have is to try something with Math.random() or with a mix of Math.random and some Date or time-related object. However, there are many modern ways to generate UUID in JavaScript and we would be exploring 2 of the most common ones today. But before we start, let us take a look at what is a UUID.
What is a UUID in JavaScript?
A UUID is a Universally Unique Identifier which is also known as GUID or Globally Unique Identifier. It is a 128-bit alpha-numeric that is unique. The UUIDs would be unique every time they are generated and hence can have many uses. As per RFC4122 version 4, UUID should be formatted as is described below:
xxxxxxxx-xxxx-Mxxx-Nxxx-xxxxxxxxxxxx
Where the allowed values of M and N are limited to 1,2,3,4 or 5.
Creating GUID/UUID in JavaScript using the Crypto API
While there are many different ways to generate UUID in JavaScript, the most modern way would be to use the built in ES6 Crypto API.
The Crypto API provides method crypto.randomUUID() that generates a v4 UUID using a cryptographically secure random number generator
Example of UUID generated using Crypto API
let uuid = crypto.randomUUID();
console.log(uuid); // for example "ab6b7b51-1c1b-4346-bc7b-d1555187ac90"
This method returns a string containing a randomly generated, 36 character long v4 UUID.
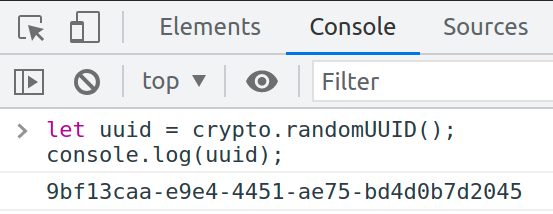
However, Please note that the support for the randomUUID() method is limited to modern browsers and might not support older browsers. Please check https://caniuse.com/mdn-api_crypto_randomuuid for the browser support list.
Creating GUID/UUID in Javascript using the npm UUID package
While using the Crypto API simply is an easy way to generate a UUID, there are times when you might want some additional features. In such cases I would recommend to use the UUID npm package which has some additional features too.
Example of UUID generated using npm UUID package
To generate a UUID using the UUID package, we need to do the following:
Firstly, install the npm package using the command:
npm install uuid
Secondly, simply import and invoke it on the page you require it as shown below:
import { v4 as uuidv4 } from 'uuid';
uuidv4(); // ⇨ 'ab6b7b51-1c1b-4346-bc7b-d1555187ac90'
The UUID npm package has many more methods that you can explore too. They claim to be more accurate and closer to the specification too and hence if you need more accurate UUIDs, you can use the package.
How to Generate UUID in React or Angular?
Well to be frank there is no need of a different way to generate UUID for React or Angular app. Either one of the above methods are good enough. For production level apps where you might need better support across browsers and more features, I would suggest that the UUID npm package might be a better option to consider.
What if crypto.getrandomvalues() is not supported by the browser?
As I mentioned earlier, currently only the modern and latest versions of the browsers have an inbuilt support of the Crypto.randomUUID() method. It could be possible that the browser your user is using might not have support for the Crypto API. In such a case you can use a polyfill for the Crypto.randomUUID method.
if (!(crypto.randomUUID instanceof Function)) {
crypto.randomUUID = function uuidv4() {
return ([1e7] + -1e3 + -4e3 + -8e3 + -1e11).replace(/[018]/g, c =>
(c ^ crypto.getRandomValues(new Uint8Array(1))[0] & 15 >> c / 4).toString(16)
);
}
}
//This polyfill was originally shared on https://stackoverflow.com/a/2117523
The polyfill method would be wrapped in an “if” condition to check for support of Crypto.randomUUID() method. If not supported by the browser, it would create a method by same name.
There can be many different ways to generate UUID in JavaScript. You can even create one of your own methods using a combination of Math.random and the Date() object etc. While there are many ways to generate UUID in JavaScript, we need to be careful of the so-called solutions available over the internet as many are based on Math.random() API and we should try to avoid solutions based on Math.random.