I know that sometimes the errors we face in JavaScript can be difficult to debug. While with all the modern tools like Chrome developer tools etc the debugging has become far easier, there still are times when we come across issues that are tricky to debug. One such issue is the “Uncaught SyntaxError: Unexpected end of input” error in JavaScript.
Let us take a look at how can we debug such issues and what might be causing them.
What causes Unexpected end of Input in JavaScript?
Well, a large number of times, the cause of the “Uncaught SyntaxError: Unexpected end of input” error in JavaScript is missing parentheses, brackets, or quotes. Let’s take a look at the example below:
var addPost = function() {
//add a new post when called
}
var editPost = function() {
//edit the post when called
}
var deletePost = function() {
//delete a post when called
var publishPost = function() {
//publish a post when called
}
While everything looks good in the code above, this was throwing the Unexpected end of input error. However, if you look carefully, you’ll notice that the deletePost
method has a missing closing bracket ( } ) which is actually causing the error.
On a javascript file with hundreds of lines of code, it can become very cumbersome to track the brackets line by line. There is an easier way to catch such errors and to avoid them too as shown in the next section.
How to fix Uncaught SyntaxError: Unexpected end of input error in JavaScript or jQuery?
Now that we know what causes this issue, the debugging method is also easy. While we can definitely look into the code and brackets and parentheses line by line, it would not be an effective method in the case of a large file. The easier way would be to make use of any tool.
One such tool I would recommend is https://jshint.com/ .
Once you visit JsHint, copy and paste your javascript code there. It would run an auto-scan of the code and list all the warnings and errors that the tool finds.
If you copy-paste the above code on JSHint, you’ll notice that it shows an ” Unmatched ‘{‘. ” warning. Once you hover over it, it would highlight the line with the deletePost method indicating that the missing bracket is in this method. Here is a screenshot that shows the issue on JsHint.
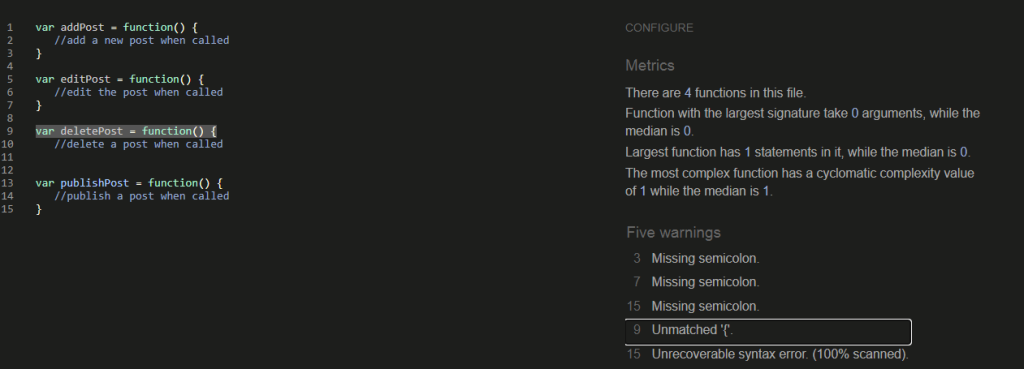
Can the error be caused due to any other reason other than missing brackets etc?
Yes! While it is true that most of the time the issue is caused due to missing parentheses, brackets, or quotes, it has been noticed that whenever we try to parse an empty response from a JSON returned by Ajax call, the Unexpected end of input error can crop up too.
In such cases, add a conditional check to see if the data returned really has something within it before making use of the data returned.
For example, consider the following Ajax call in jQuery:
$.ajax({
url: url,
...
success: function (response) {
if(response.data.length == 0){
// this condition would prevent a blank json from being parsed
// Empty response returned, maybe show an error etc
}else{
//Parse the JSON and use it
}
}
});
In the above code, you can notice that once we receive the response, we check if the length of the data returned is 0 i.e if the data returned is empty then maybe write a handler method or show an error to the user, otherwise, if there is data then parse it and use it.
To Summarize, the causes of Uncaught SyntaxError: Unexpected end of input error can be:
- Missing Parentheses in the code
- Missing Bracket in the code
- Missing quote in the code, for string etc
- Parsing an empty JSON
As I mentioned earlier, the “Uncaught SyntaxError: Unexpected end of input” error in JavaScript can be caused due to various reasons, however, the majority of the time it is because of missing parentheses, bracket, quote or because of parsing an empty JSON. However, an easy way to spot and avoid such issues is to run your javaScript through a validator like jsHint.com. If you are using webpack then you can even configure JSLinting or ESlint in your build process which would highlight such warnings and errors during the build time itself. Hope you would this article useful. Let us know in the comments!